For more details on this content, please review the step-by-step guide and frequently asked questions.
How To Code a Simple Webpage with HTML
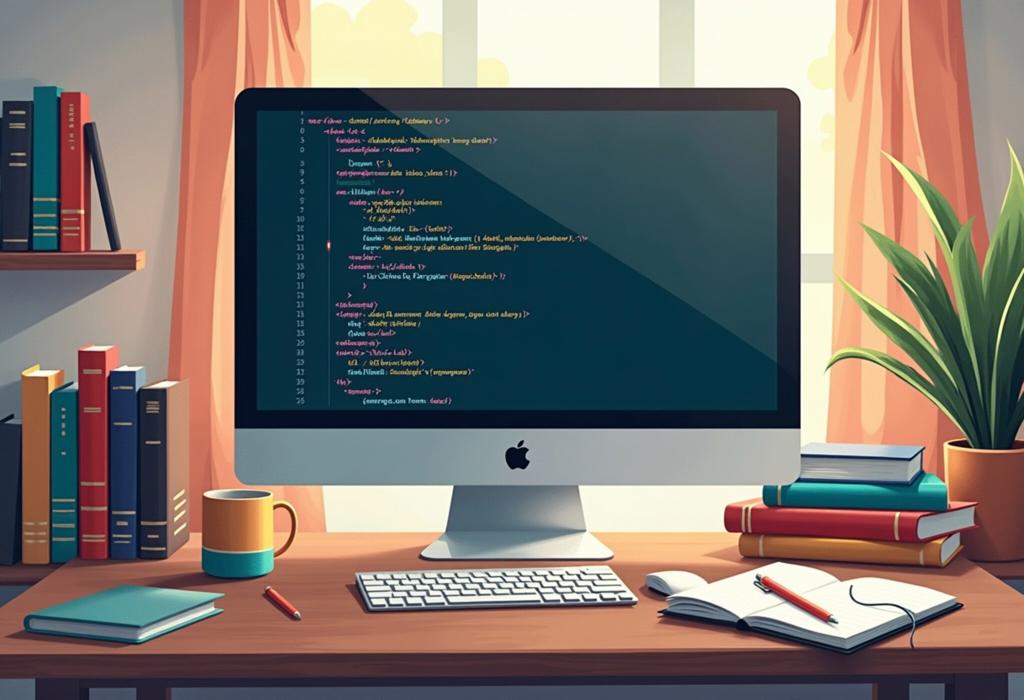
Step-by-Step Guide
Understanding HTML
HTML stands for HyperText Markup Language. It's the standard markup language used to create web pages. It structures the content on the web and allows you to define elements like headings, paragraphs, links, and images.
Setting Up Your Environment
Choose a text editor to write your HTML code. Popular choices include Visual Studio Code, Sublime Text, and Notepad++. Ensure that you have a web browser (like Chrome, Firefox, or Safari) to view your webpage.
Creating Your HTML File
Open your text editor and create a new file. Save it with a .html extension, for example, 'index.html'. This tells the computer that this file is an HTML document.
Writing the Basic Structure
Start by writing the basic HTML structure. This includes the <!DOCTYPE html> declaration, an <html> tag, a <head> section for metadata, and a <body> section for content. Here's a simple template: ```html <!DOCTYPE html> <html> <head> <title>Your Page Title</title> </head> <body> <h1>Welcome to My Webpage</h1> <p>This is my first web page!</p> </body> </html> ```
Adding Headings and Paragraphs
Use the <h1> to <h6> tags for headings (h1 is the largest, h6 is the smallest). For regular text, use the <p> tag to make paragraphs. For example: ```html <h2>About Me</h2> <p>I love coding and creating websites!</p> ```
Inserting Links
To create a webpage link, use the <a> tag. The href attribute defines the URL of the page the link goes to. Example: ```html <a href='https://www.example.com'>Visit Example</a> ```
Adding Images
To insert images, use the <img> tag with the src attribute for the image source and alt for alternative text. Example: ```html <img src='image.jpg' alt='Description of the image'> ```
Creating Lists
Use <ul> for unordered lists and <ol> for ordered lists. List items are wrapped in <li> tags. Example: ```html <ul> <li>Item 1</li> <li>Item 2</li> </ul> ```
Adding Styles with CSS
To enhance the look of your webpage, you can add styles using CSS. Inline styles can be added using the `style` attribute in HTML tags, or you can link to a separate CSS file. Example: ```html <style> body { font-family: Arial, sans-serif; } </style> ```
Previewing Your Work
Open your .html file in a web browser to see your webpage in action. Right-click the file and choose the option to open it with your preferred browser.
Making Adjustments
As you preview your webpage, you may find that you want to change some text or styles. Go back to your text editor, make the changes, save the file, and refresh the browser to see your updates.
Expanding Your Skills
Once you're comfortable with the basics of HTML, consider learning more about CSS for styling and JavaScript for interactivity. Practice by creating multiple pages or more complex layouts.